Learn how to create a circular gradient in Shadertoy, using the GLSL programming language.
Constants
Define two colour constants.
const vec3 COL1 = vec3(0.06, 0.4, 0.07);
const vec3 COL2 = vec3(0.68, 0.85, 0.68);
And a value for the edge smoothing.
const float SMOOTHING = 128.0;
Circle function
Create a circle function to calculate the distance to the centre of a circle.
float Circle(vec2 pos, float radius)
{
return length(pos) - radius;
}
Aspect ratio
Calculate the aspect ratio inside the main function using the resolution.
float aspect = 1.0 / min(iResolution.x, iResolution.y);
UV coordinates
Calculate aspect ratio corrected UV coordinates.
vec2 uv = fragCoord * aspect;
Position
Create a position variable, and set it to the UV coordinates. Offset it to the centre of the screen using half of the resolution and the aspect ratio.
vec2 pos = uv;
pos -= aspect * iResolution.xy / 2.0;
Create circle
Create a circle using the position and a radius value which controls the size of the circle.
float radius = 0.25;
float c = Circle(pos, radius);
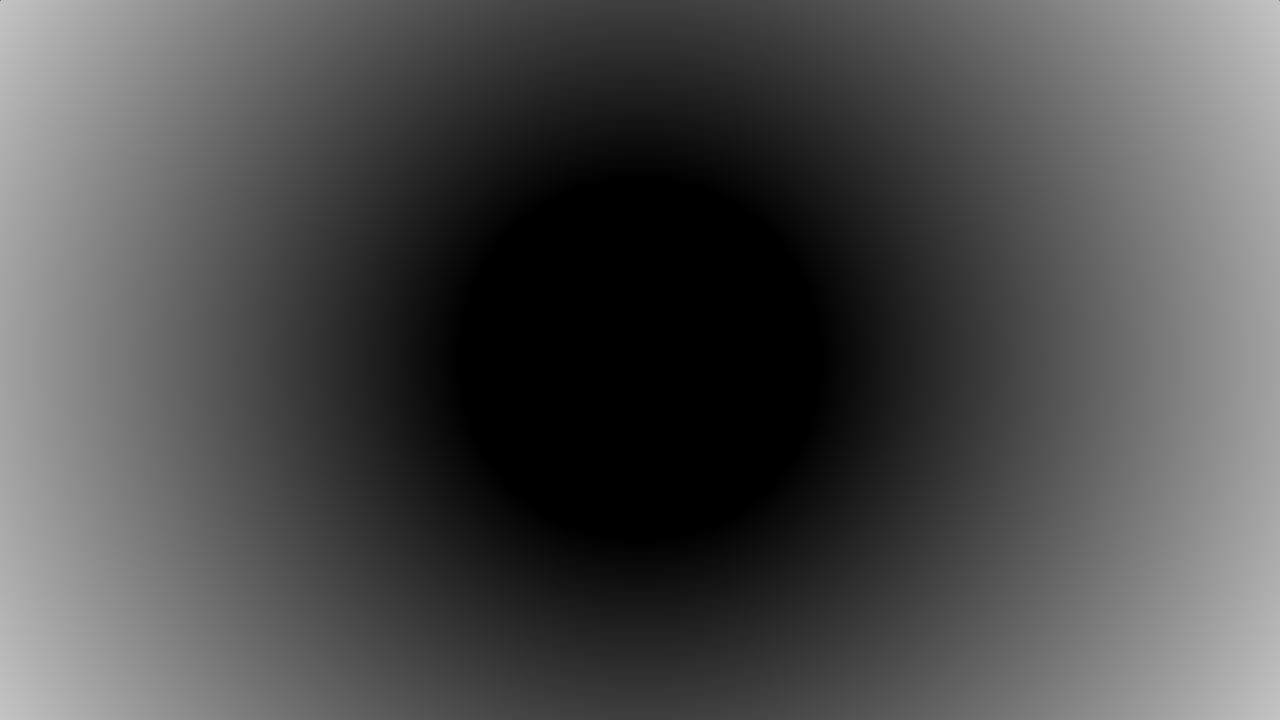
Edge smoothing
Smooth the edges of the circle using the smoothing value from earlier and the aspect ratio.
float s = SMOOTHING * aspect;
c = smoothstep(s, -s, c);
Test
You can see the size of the circle by setting the RGB of the output fragment to the circle distance value.
fragColour.rgb = vec3(c);
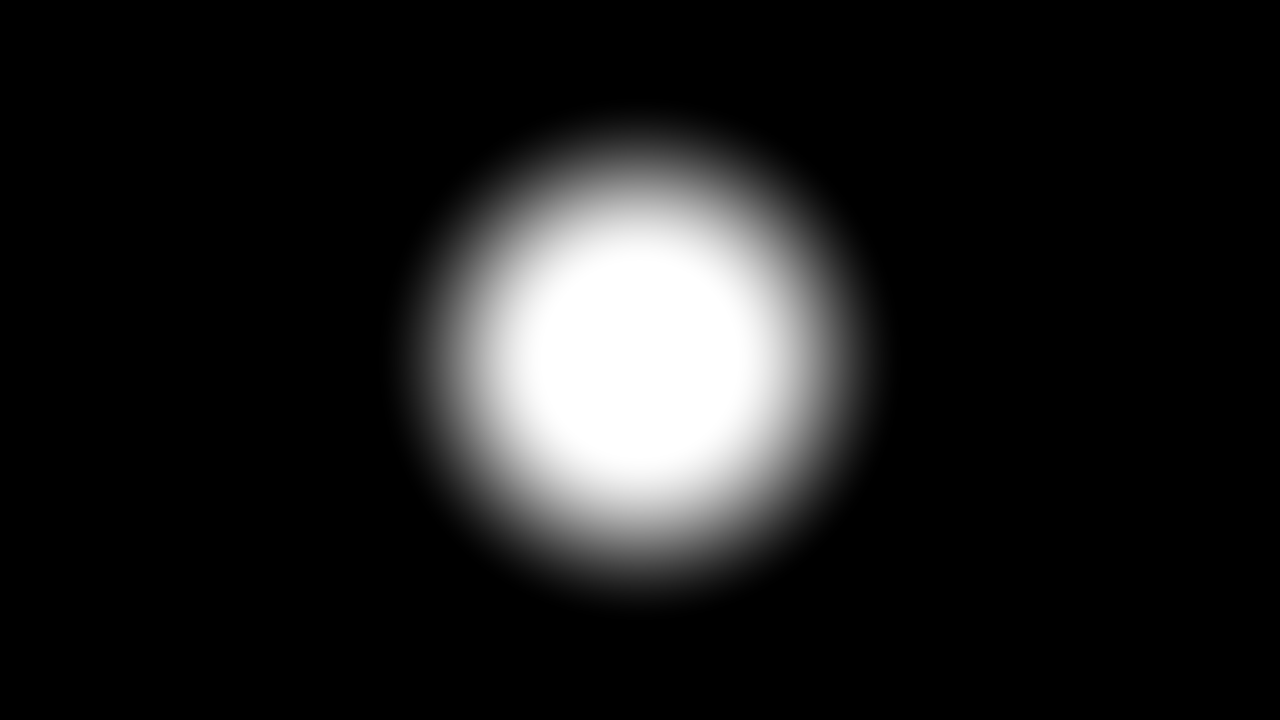
Mix
Mix the two colours we defined earlier with the circle distance value. Multiply the result against the circle distance value, so that the gradient is only created inside the circle.
fragColour.rgb = mix(COL1, COL2, c) * c;
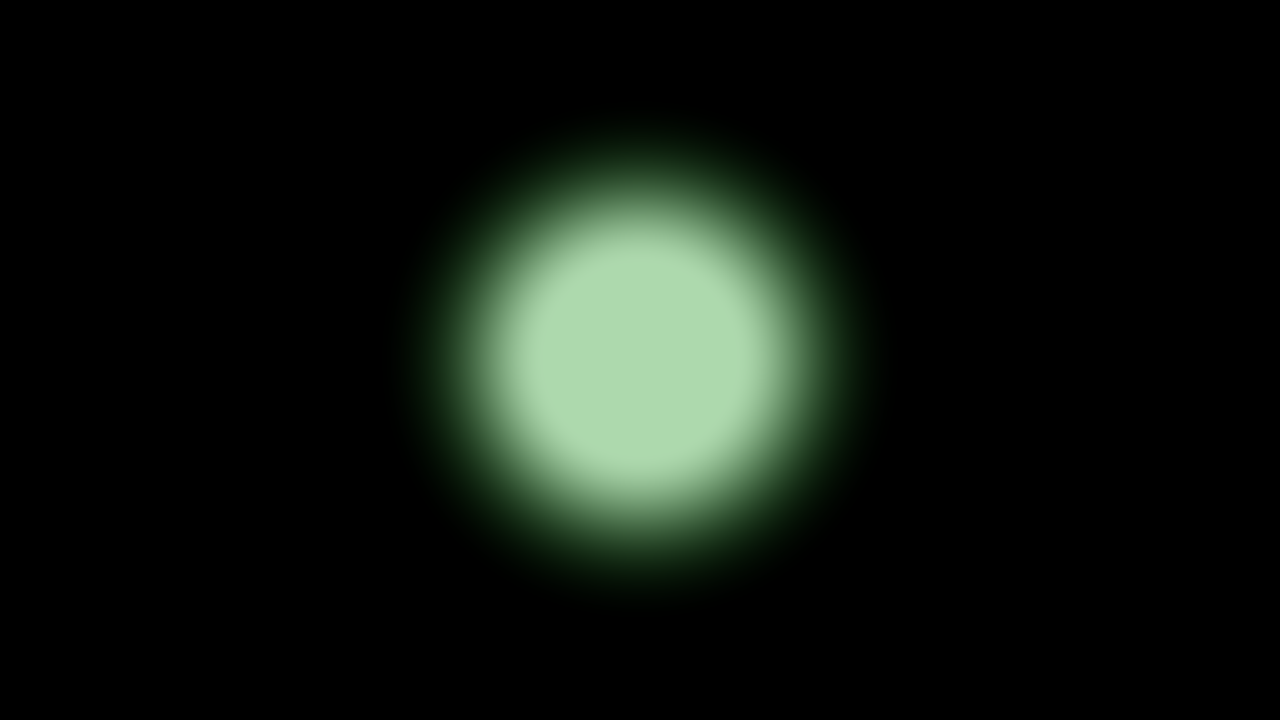
Alpha
Set the alpha of the output fragment value.
fragColour.a = 1.0;
Animate
Optionally animate the circle’s radius using time and a sine wave.
float t = sin(iTime) * 0.5 + 0.5;
float radius = mix(0.0, 0.6, t);
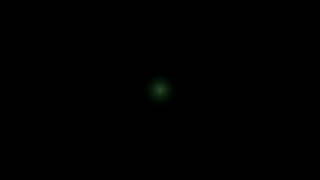
Shadertoy
Full Shadertoy code and demo available here.
Conclusion
Thank you for reading this tutorial. Let me know in the comments section if you enjoyed it, or have any questions!
For more gradient tutorials, check out:
2 responses to “Creating a circular gradient in Shadertoy”
Thanks 😀
LikeLiked by 1 person
Thanks for visiting!
LikeLiked by 1 person