Learn how to create a rotated rectangle transition in Shadertoy using the GLSL programming language. Useful in video games and movies when changing scenes.
Introduction
For this tutorial I will use these fantastic images from Astro Planes Art.
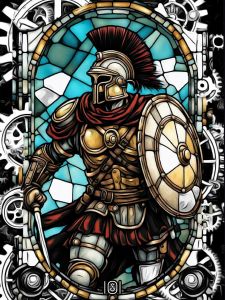
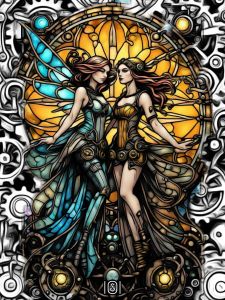
Constants
First define PI and TWO_PI.
#define PI 3.14159265359
#define TWO_PI PI * 2.0
Then define a constant for smoothing the rectangle’s edges.
const float EDGE_SMOOTHING = 1.0;
Next define a constant which controls the transition speed.
const float SPEED = 0.64;
Lastly create a constant for the background colour.
const vec4 BACKGROUND = vec4(0.0, 0.0, 0.0, 1.0);
Rotate function
Create a rotation function, to rotate the UV coordinates.
vec2 RotateUV(vec2 uv, float rotation)
{
return vec2(
cos(rotation) * uv.x + sin(rotation) * uv.y,
cos(rotation) * uv.y - sin(rotation) * uv.x
);
}
Rectangle function
Define a 2D SDF function for a rectangle. Obtained from here.
float Rectangle(in vec2 pos, in vec2 size)
{
vec2 d = abs(pos) - size;
return length(max(d, 0.0)) + min(max(d.x, d.y), 0.0);
}
UV coordinates
Define the UV coordinates inside the main function.
// Normalised pixel coordinates (from 0 to 1)
vec2 uv = fragCoord / iResolution.xy;
Aspect ratio
Calculate the aspect ratio using the resolution.
float aspect = 1.0 / min(iResolution.x, iResolution.y);
Progress
Define a progress value which uses the current time and speed constant. The value will move back and forth in the 0 to 1 range.
float progress = sin(iTime * SPEED) * 0.5 + 0.5;
Offset
Offset UV coordinates towards the centre, then rotate using the TWO_PI value.
uv -= 0.5;
uv = RotateUV(uv, mix(0.0, TWO_PI, progress));
uv += 0.5;
Sync
Sync the texture switching with the scaling. So the texture switching, scaling and rotation occur at the same time.
float p = abs(progress - 0.5) * 2.0;
Create rectangle
Create the rectangle by defining a position and size using the ‘p’ variable. Mix the minimum size with the maximum size. This will animate the size.
// Rectangle position
vec2 pos = uv - 0.5;
// Animate the rectangle size
vec2 size = vec2(mix(0.0, 0.5, p));
// Create rectangle
float b = Rectangle(pos, size);
Edge smoothing
Smooth the edges of the rectangle. Define a smooth value using the smoothing constant from earlier and the aspect ratio. Use the smoothstep function with the rectangle distance and the smooth value.
float s = EDGE_SMOOTHING * aspect;
b = smoothstep(s, -s, b);
Textures
Add two textures to the first two channels in Shadertoy. Then get the colours from both of the textures.
vec4 from = texture(iChannel0, uv);
vec4 to = texture(iChannel1, uv);
Output
Mix both texture colours based on the result of the step function. The step function is checking if the progress value is less than 0.5. If it is, then the first texture will be used. Else the second texture will be used.
vec4 col = mix(from, to, step(0.5, progress));
Finally mix the current colour with the background colour based on the circle size.
fragColour = mix(BACKGROUND, col, b);
Easing
Optionally apply an easing function to the ‘p’ variable.
// https://easings.net/#easeInOutCubic
float EaseInOutCubic(float x)
{
return x < 0.5 ? 4.0 * x * x * x : 1.0 - pow(-2.0 * x + 2.0, 3.0) / 2.0;
}
Using the easing function:
p = EaseInOutCubic(p);
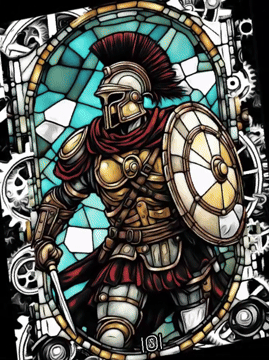
Shadertoy
Full Shadertoy code and demo available here.
Conclusion
Thank you for reading this tutorial. Let me know in the comments section if you enjoyed it, or have any questions!
8 responses to “Rotated rectangle Shadertoy transition tutorial”
Hell yea!!
I love this!!!
SM101
LikeLiked by 1 person
Thanks, it’s looking great with your art 🙂
LikeLiked by 1 person
It sure is man, I envy you coder guys I understand your work I just really suck at it, guys new to never let me set up the ciscos 😂
I told you use what you want anytime D
SM101
LikeLiked by 1 person
Will do 😀 And if you get bored, and want to learn some more coding, you know where the tutorials are!
LikeLiked by 1 person
These days I do everything I do on my android phone I live OTG on 12 volt power system. Have not even turned my laptop on in 7 years…
I have plenty to keep me busy but I do know where to find ya!! Have a great day Dragon!!
LikeLiked by 1 person
That’s pretty cool, sounds like you will be able to survive the apocalypse. I like my android phone to, and have done coding on it before. But my PC / laptop is better for productivity for me.
Have an awesome day!
LikeLike
Thanks 😀
LikeLiked by 1 person
Thanks for visiting Anita 🙂
LikeLiked by 1 person